swagger2
依赖jar包
引入包 |
版本 |
jdk |
1.8 |
spring boot |
2.7.2 |
springfox-swagger2 |
3.0.0 |
swagger-annotations |
1.5.24 |
swagger-models |
1.5.24 |
knife4j-spring-ui |
2.0.8 |
spring-boot-autoconfigure |
2.7.2 |
使用
添加依赖
1 2 3 4 5
| <dependency> <groupId>cn.allbs</groupId> <artifactId>allbs-swagger</artifactId> <version>1.1.8</version> </dependency>
|
1
| implementation 'cn.allbs:allbs-swagger:1.1.8'
|
1
| implementation("cn.allbs:allbs-swagger:1.1.8")
|
开启swagger功能
启动类添加@EnableAllbsSwagger2
注解
过滤uri不映射出来
如果使用了通用包中的@AllbsResponseAdvice
包装了返回结果则需要添加以下配置
1 2 3 4
| ignore: urls: - /swagger-resources - /v2/api-docs
|
swagger2配置说明(根据自己实际情况配置)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| swagger: base-package: cn.allbs.swagger.controller title: 标题dev description: 标题dev version: 1.0 term-service-url: termUrl host: localhost contact: name: 这是name url: 这是url email: [email protected] auth: token ignore-prefix: - /user
|
效果说明
没有配置在ignore-prefix中的效果
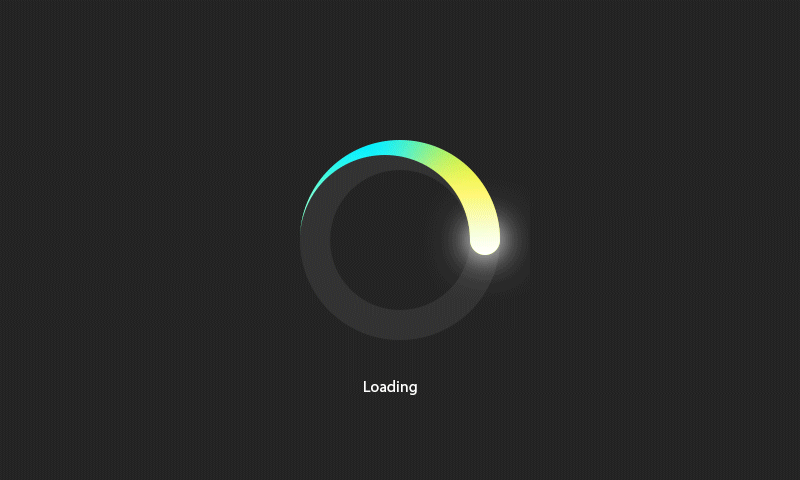
配置中存在ignore-prefix中的效果
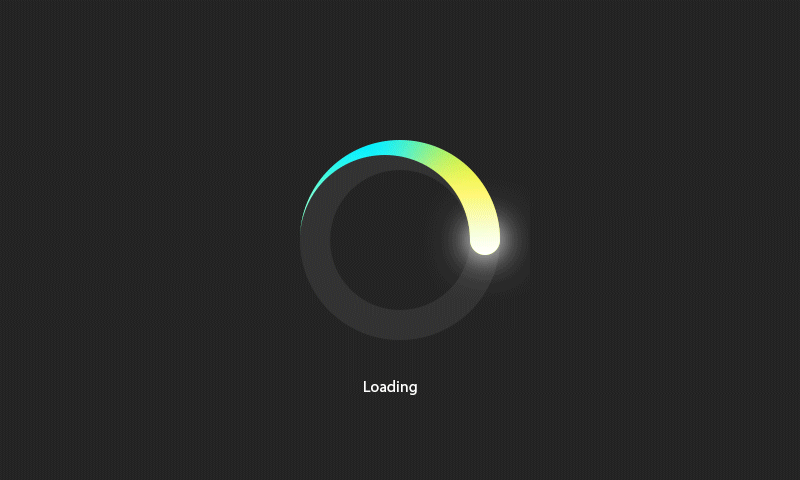
备注
swagger配置设置为仅在profiles为dev以及test的配置中生效,其他一律不生效
访问地址
http://{ip}:{port}/doc.html
其他说明
如果服务配置端口为6666
,6667
等部分端口ui界面无法访问,暂未查出原因
代码示例
1 2 3 4 5 6 7 8 9 10 11 12 13
| @Data @ApiModel(value = "swagger类") public class SwaggerEntity {
@ApiModelProperty(value = "时间") private LocalDateTime time;
@ApiModelProperty(value = "名称") private String name;
@ApiModelProperty(value = "计数") private Integer count; }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| package cn.allbs.swagger.controller;
import cn.allbs.common.utils.R; import cn.allbs.swagger.entity.SwaggerEntity; import io.swagger.annotations.*; import lombok.RequiredArgsConstructor; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController;
import java.time.LocalDateTime;
@Api(tags = "这是类的作用", value = "没啥用的") @RestController @RequestMapping("user") @RequiredArgsConstructor public class TestNoAuthController {
@ApiOperation(value = "方法的用途", notes = "方法的备注说明") @ApiImplicitParams({ @ApiImplicitParam(name = "名称", value = "这是名称的注释", required = true, paramType = "query", dataType = "String", dataTypeClass = String.class, defaultValue = "这是默认值"), @ApiImplicitParam(name = "计数", value = "这是技术的注释", required = false, paramType = "query", dataType = "Integer", dataTypeClass = Integer.class, defaultValue = "22"), }) @ApiResponses({ @ApiResponse(code = 200, message = "名称", reference = "name", response = String.class), @ApiResponse(code = 200, message = "时间", reference = "time", response = LocalDateTime.class), @ApiResponse(code = 200, message = "计数", reference = "count", response = Integer.class) }) @GetMapping("swaggerTest") public R<SwaggerEntity> swaggerTest(String name, Integer count) { SwaggerEntity swaggerEntity = new SwaggerEntity(); swaggerEntity.setName(name); swaggerEntity.setTime(LocalDateTime.now()); swaggerEntity.setCount(count); return R.ok(swaggerEntity); } }
|
swagger3
依赖jar包
引入包 |
版本 |
jdk |
1.8 |
spring boot |
2.7.2 |
knife4j-springdoc-ui |
3.0.3 |
spring-boot-autoconfigure |
2.7.2 |
springfox-boot-starter |
3.0.0 |
使用
添加依赖
maven
1 2 3 4 5
| <dependency> <groupId>cn.allbs</groupId> <artifactId>allbs-swagger3</artifactId> <version>2.0.1</version> </dependency>
|
Gradle
1
| implementation 'cn.allbs:allbs-swagger3:2.0.1'
|
开启swagger功能
启动类添加@EnableAllbsSwagger3
注解
过滤uri不映射出来
如果使用了通用包中的@AllbsResponseAdvice
包装了返回结果则需要添加以下配置
1 2 3
| ignore: urls: - /v3/api-docs
|
swagger2配置说明(根据自己实际情况配置)
1 2 3 4 5 6 7 8 9 10 11 12
| swagger: title: springDoc文档 description: 这是springdoc文档说明 version: 1.0 securitySchemes: token: type: APIKEY in: HEADER name: token servers: - url: http://127.0.0.1:8888 description: 本地服务器
|
备注
1 2 3 4 5 6 7 8
| springdoc: api-docs: enabled: false packages-to-scan: cn.allbs.swagger.controller paths-to-match: /user/**
|
访问地址
http://{ip}:{port}/doc.html
使用示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| package cn.allbs.swagger.entity;
import io.swagger.v3.oas.annotations.media.Schema; import lombok.Data;
import java.time.LocalDateTime;
@Data @Schema(title = "swagger类", name = "SwaggerEntity") public class SwaggerEntity {
@Schema(description = "时间", name = "time", implementation = LocalDateTime.class, example = "2022-08-09'T'12:00:00") private LocalDateTime time;
@Schema(description = "名称", name = "name", implementation = String.class, example = "张三") private String name;
@Schema(description = "计数", name = "count", implementation = Integer.class, example = "12") private Integer count; }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| package cn.allbs.swagger.controller;
import cn.allbs.common.utils.R; import cn.allbs.swagger.entity.SwaggerEntity; import io.swagger.v3.oas.annotations.Operation; import io.swagger.v3.oas.annotations.Parameter; import io.swagger.v3.oas.annotations.Parameters; import io.swagger.v3.oas.annotations.enums.ParameterIn; import io.swagger.v3.oas.annotations.media.Content; import io.swagger.v3.oas.annotations.media.ExampleObject; import io.swagger.v3.oas.annotations.media.Schema; import io.swagger.v3.oas.annotations.responses.ApiResponse; import io.swagger.v3.oas.annotations.responses.ApiResponses; import io.swagger.v3.oas.annotations.tags.Tag; import lombok.RequiredArgsConstructor; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController;
import java.time.LocalDateTime;
@Tag(name = "B类") @RestController @RequestMapping("user") @RequiredArgsConstructor public class TestNoAuthController {
@Operation(summary = "B类A方法") @Parameters({ @Parameter(name = "name", description = "这是名称的注释", required = true, schema = @Schema(implementation = String.class), in = ParameterIn.QUERY), @Parameter(name = "count", description = "这是计数的注释", required = false, schema = @Schema(implementation = Integer.class), in = ParameterIn.QUERY), }) @ApiResponses({ @ApiResponse(responseCode = "200", description = "查询成功", content = {@Content(mediaType = "application/json", schema = @Schema(implementation = SwaggerEntity.class), examples = {@ExampleObject(value = "{\n" + " \"code\": 200,\n" + " \"success\": true,\n" + " \"msg\": \"操作成功\",\n" + " \"data\": {\n" + " \"time\": \"2022-08-09T15:44:40.991\",\n" + " \"name\": \"xxx\",\n" + " \"count\": 123\n" + " }\n" + "}")})}) }) @GetMapping("swaggerTest") public R<SwaggerEntity> swaggerTest(String name, Integer count) { SwaggerEntity swaggerEntity = new SwaggerEntity(); swaggerEntity.setName(name); swaggerEntity.setTime(LocalDateTime.now()); swaggerEntity.setCount(count); return R.ok(swaggerEntity); } }
|
swagger2及swagger3注解对应关系
SpringFox |
SpringDoc |
@Api |
@Tag |
@ApiIgnore |
@Parameter(hidden = true)or@Operation(hidden = true)or@Hidden |
@ApiImplicitParams |
@Parameters |
@ApiImplicitParam |
@Parameter |
@ApiModel |
@Schema |
@ApiModelProperty |
@Schema |
@ApiOperation(value = “foo”, notes = “bar”) |
@Operation(summary = “foo”, description = “bar”) |
@ApiParam |
@Parameter |
@ApiResponse(code = 404, message = “foo”) |
ApiResponse(responseCode = “404”, description = “foo”) |